In this lesson, we will learn different ways(10 Ways to Get User ID) to find the user ID of WordPress users.
But before we look at how to find these IDs, let me first show you how you can get detailed information about a user using their ID. To keep it simple, the “get_user_by()” function is the best way to do this.
$user = get_user_by( ‘id’, 1 ); // 1 is a user ID
echo $user->first_name; // let’s pring the first name of this user
1. In URL when you edit the user
This method is really easy, but it has some downsides. First, you need to be logged in, and second, you can’t get your own ID using this method.
Here’s what you need to do:
- Log in to your WordPress admin.
- Go to “Users” and click on “All users.”
- Select the user you want and go to their profile.
- Check the web address (URL) of the page.
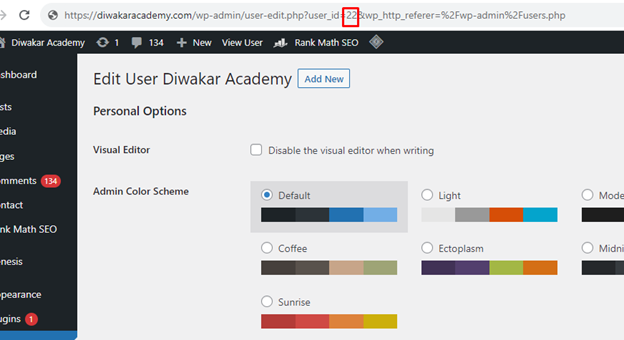
2. Add a custom column with user ID
Another way to find a particular user’s ID without writing any code is by adding a new column to the Users table, like this:
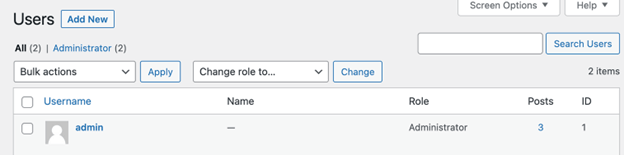
To show the column, just put this code into your functions.php file:
// register the column add_filter( 'manage_users_columns', 'dwakar_academy_user_id_column' ); function dwakar_academy_user_id_column( $columns ) { $columns[ 'user_id' ] = 'ID'; return $columns; } // populate the column with data add_action( 'manage_users_custom_column', 'dwakar_academy_column_content', 10, 3); function dwakar_academy_column_content( $value, $column_name, $user_id ) { if( 'user_id' === $column_name ) { return $user_id; } return $value; } // CSS for the column add_action( 'admin_head-users.php', 'dwakar_academy_column_style' ); function dwakar_academy_column_style(){ echo '<style>.column-user_id{ width: 5% }</style>'; }
3-4. Get current user ID in WordPress
get_current_user_id()
The easiest way to get the ID of the user who is currently logged in and looking at a particular page (where the code is active) is:
$current_user_id = get_current_user_id();
Another method is to use the “wp_get_current_user()” function. This is handy when you want to get not only the ID of the current user but also some extra information like their first name or email address.
$current_user = wp_get_current_user(); // current user ID $current_user_id = $current_user->ID; // current user email $current_user_email = $current_user->user_email; // current user login (username) $current_user_login = $current_user->user_login; $current_user_name = array( 'first' => $current_user->first_name, 'last' => $current_user->last_name );
There’s also a function called “get_currentuserinfo(),” but it’s outdated and no longer recommended, so avoid using it.
5. Get user ID by email
It’s pretty straightforward. You can use the “get_user_by()” function that I mentioned earlier.
For example, if you want to find a user’s ID by their email, you can do this:
$user = get_user_by('email', '[email protected]'); $user_id = $user->ID;
And if you want to find a user’s email by their ID, you can use “get_user_by()” again:
$user = get_user_by('id', 54); echo $user->user_email;
Alternatively, you can also use “get_userdata()” like this:
$user = get_userdata(54); echo $user->user_email;
Both methods will give you the user’s email when you know their ID.
6. Get user ID by username (login)
In the next example, the “diwakar” you see as the second part is the username.
Here’s the code:
$user = get_user_by('login', 'diwakar'); $user_id = $user->ID;
Now, if you want to get the username when you know the user’s ID, you can do it. You can use either “get_userdata()” or “get_user_by(‘id’, …)” just like we did in the previous examples with emails.
7-8. Get user ID by first name or by last name
Here’s how you can print the IDs of all users with the first name “diwakar”:
global $wpdb; $users = $wpdb->get_results( " SELECT user_id FROM $wpdb->usermeta WHERE meta_key = 'first_name' AND meta_value = 'diwkar' " ); if( $users ) { foreach ( $users as $user ) { echo '<p>' . $user->user_id . '</p>'; } } else { echo 'There are no users with the specified first name.'; } ```
The reason we use `get_results()` instead of `get_var()` here is that there might be multiple users with the same name.
To print the IDs of all users with the last name “Academy,” you can use this code:
global $wpdb; $users = $wpdb->get_results( "SELECT user_id FROM $wpdb->usermeta WHERE meta_key = 'last_name' AND meta_value = 'Academy'" ); if( $users ) { foreach ( $users as $user ) { echo '<p>' . $user->user_id . '</p>'; } } else { echo 'There are no users with the specified last name.'; } ```
This code can be adapted to find a user’s ID based on any user metadata. Just replace `meta_key` and `meta_value` with the specific metadata you’re looking for.
Additionally, you can retrieve a user’s first and last names or any other user metadata using the `get_user_meta( $id, $meta_key, true)` function.
9. Get author ID by post ID?
In this situation, you can easily find the user’s ID from a WP_Post object. It’s a straightforward process.
$my_post = get_post( $id ); // $id - Post ID echo $my_post->post_author; // print post author ID
10. Get customer ID from WooCommerce order?
You can find the customer’s ID from an order in two different ways. The first way is by getting the customer ID from the order’s metadata like this:
$customer_id = get_post_meta(541, ‘_customer_user’, true); // 541 is your order ID
The second way is to use the WC_Order object. Please note that this method only works for WooCommerce versions 3.0 and above. Here’s how you can do it:
$order = wc_get_order(541); // 541 is your order ID
$customer_id = $order->get_customer_id(); // You can also use $order->get_user_id(); it’s the same thing. Both methods will help you get the customer’s ID from an order.
Related Articles
Leave a Reply