In this post I am going to show how to create Custom Post Type Ajax Filter. Following requirement given below.
On Back-end
- Create Custom Post Type(CPT) as Movie
- Create taxonomy as Movie Categories(terms would be bollywood, hollywood, tollywood, pollywood) for Movie CPT
- Create Custom Field as Popularity with the values from 1 to 5. Which can be set manually from backend. You can use ACF to create a Popularity Custom field.
On Front-end
- Create select fields of terms with all terms created within Movie Categories taxonomy
- Create select fields of Popularity with 1 to 5 values
- Pull the popular movie that is Rated as 5 in back-end
- The movie listing should change based on selections of Rating, Terms and Both
- Code should work only with AJAX on changing of Popularity and Movie Categories in drop down
Step 1. Create a child theme
twentytwenty-child/style.css
/* Theme Name: Twenty Twenty Child Theme URI: http://example.com/twenty-fifteen-child/ Description: Twenty Twenty Child Theme Author: John Doe Author URI: http://example.com Template: twentytwenty Version: 1.0.0 License: GNU General Public License v2 or later License URI: http://www.gnu.org/licenses/gpl-2.0.html Tags: light, dark, two-columns, right-sidebar, responsive-layout, accessibility-ready Text Domain: twentytwenty */
twentytwenty-child/functions.php
<?php add_action( 'wp_enqueue_scripts', 'my_theme_enqueue_styles' ); function my_theme_enqueue_styles() { $parenthandle = 'parent-style'; // This is 'twentyfifteen-style' for the Twenty Fifteen theme. $theme = wp_get_theme(); wp_enqueue_style( $parenthandle, get_template_directory_uri() . '/style.css', array(), // if the parent theme code has a dependency, copy it to here $theme->parent()->get('Version') ); wp_enqueue_style( 'child-style', get_stylesheet_uri(), array( $parenthandle ), $theme->get('Version') // this only works if you have Version in the style header ); }
Step 2. Create custom post type
add given below code in twentytwenty-child/functions.php
add_action('init','register_custom_post_types'); function register_custom_post_types(){ register_post_type('movie',[ 'labels' => [ 'name' => 'Movie', 'singular_name' => 'Movie', 'menu_name' => 'Movies', ], 'public' => true, 'publicly_queryable' =>true, 'menu_icon' => 'dashicons-format-video', 'has_archive' =>true, 'rewrite' => ['slug' => 'movie'], 'supports' => [ 'title', 'editor', 'thumbnail', ], ] ); }
Step 3. Create custom taxonomy
add given below code in twentytwenty-child/functions.php
add_action('init','register_taxonomies'); function register_taxonomies(){ register_taxonomy('movie_type',['movie'], [ 'hierarchical' => true, 'labels' => [ 'name' => __('Categories'), 'singular_name' => __('Category'), 'menu_name' => __('Categories'), ], 'show_ui' => true, 'show_admin_column' => true, 'rewrite' => ['slug' => __('type')], ] ); }
Step 4. Add ACF Plugin
Add ACF Plugin and create a custom rating field
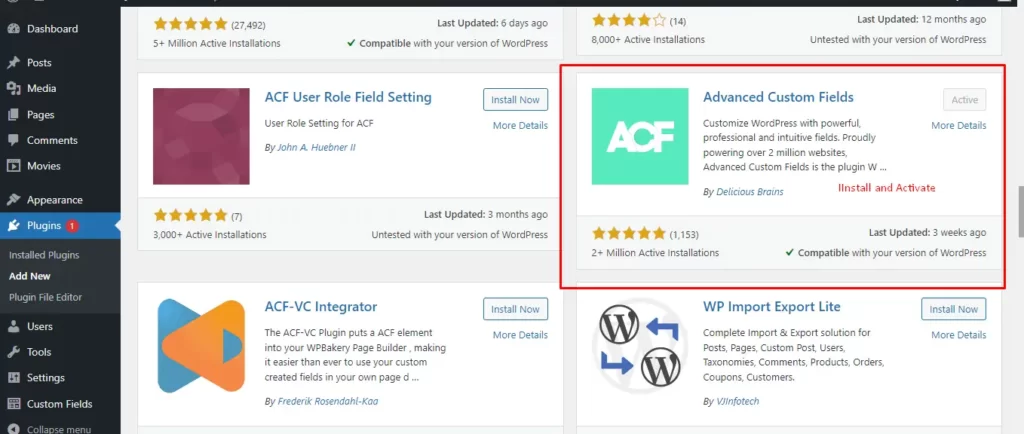
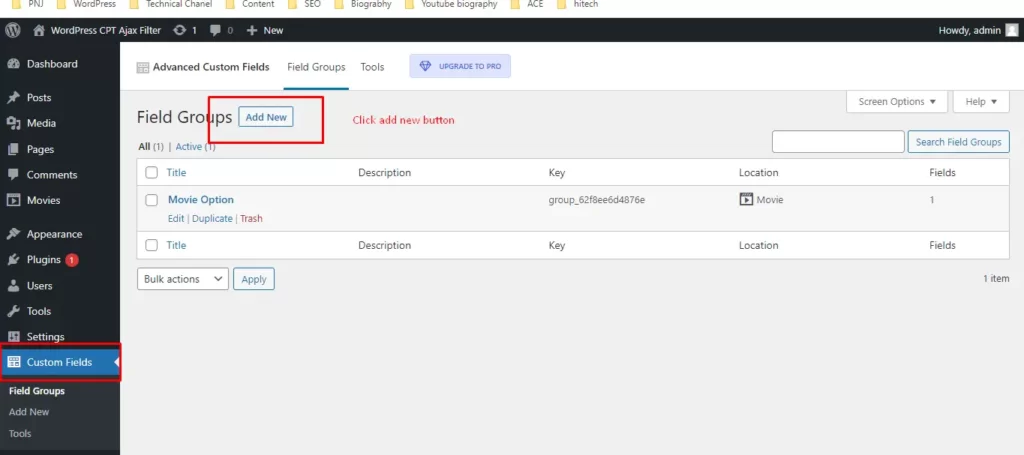
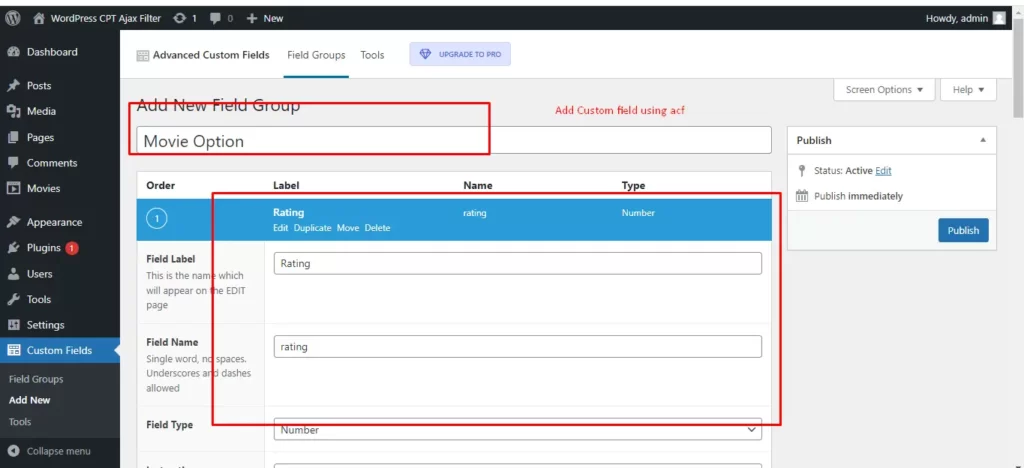
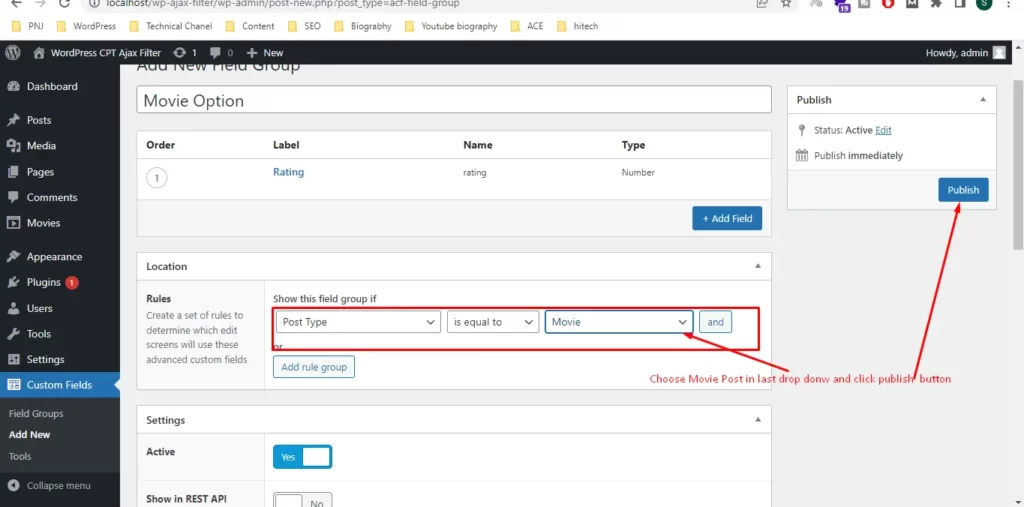
Step 5. Display Movie List
create a template file for movie list
twentytwenty-child/template-movies.php
<?php /*Template Name: Template Movies*/ get_header(); $args =[ 'post_type' => 'movie', 'posts_per_page' => -1, ]; $movies = new WP_Query($args); ?> <main> <div class="movie_container" style="width: 80%; margin: 0 auto;"> <?php if($movies->have_posts()): ?> <div class="js-movies row"> <?php while ($movies->have_posts()): $movies->the_post(); get_template_part('template-parts/loop','movie'); endwhile; ?> </div> <?php endif; ?> </div> </main> <?php get_footer(); ?>
twentytwenty-child/template-parts/loop-movie.php
<div class="column column-4"> <?php if(has_post_thumbnail()): ?> <picture><img width="500" height="250" src="<?php the_post_thumbnail_url(); ?>" alt="<?php the_title(); ?>"> </picture> <?php endif; ?> <h4><?php the_title(); ?></h4> <?php $cats = get_the_terms(get_the_ID(),'movie_type'); $rating = get_field('rating'); if(!empty($cats) || !empty($rating)): ?> <ul> <?php if(!empty($cats)): ?> <li> <strong>Category: </strong> <?php foreach ($cats as $cat){ echo "<span>$cat->name</span>"; } ?> </li> <?php endif; if(!empty($rating)):?> <li> <strong>Rating: </strong> <?php echo $rating; ?> </li> <?php endif; ?> </ul> <?php endif; ?> </div>
add given css in twentytwenty-child/style.css file
.movie_container{ width: 80%; margin: 0 auto; } /* Default Settings */ .row, .column { box-sizing: border-box; } .row:before, .row:after { content: ''; display: table; } .row:after { clear: both; } .column { position: relative; display: block; } /* Mobile Devices Styling */ .column-1, .column-2, .column-3, .column-4, .column-5, .column-6, .column-7, .column-8, .column-9, .column-10, .column-11, .column-12 { width: auto; float: none; } /* General Styling */ body { padding: 10px; } .row { margin-bottom: 0; } .column { color: #fff; padding: 20px; min-height: 30px; margin-bottom: 10px; margin-right: 10px; } .row:last-child .column:last-child { margin-bottom: 0; } .column-1 { background-color: #54B2B6; } .column-2 { background-color: #DB4251; } .column-3 { background-color: #8AAF6D; } .column-4 { background-color: #F5B939; } .column-5 { background-color: #927F99; } .column-6 { background-color: #96AFFF; } .column-7 { background-color: #54B2B6; } .column-8 { background-color: #1A97C0; } .column-9 { background-color: #777777; } .column-10 { background-color: #259E78; } .column-11 { background-color: #1A97C0; } .column-12 { background-color: #54B2B6; } /* Larger Screens Styling */ @media only screen and (min-width: 768px) { .column { position: relative; float: left; margin-bottom: 0; margin-top: 10px; } .row { margin-bottom: 10px; } .row .row:last-child { margin-bottom: 0; } .column-1 { width: 6.86666666667%; } .column-2 { width: 15.3333333333%; } .column-3 { width: 23.8%; } .column-4 { width: 32.2666666667%; } .column-5 { width: 40.7333333333%; } .column-6 { width: 49.2%; } .column-7 { width: 57.6666666667%; } .column-8 { width: 66.1333333333%; } .column-9 { width: 74.6%; } .column-10 { width: 83.0666666667%; } .column-11 { width: 91.5333333333%; } .column-12 { width: 100%; } }
Step 6. Add drop down Ajax Filter
twentytwenty-child/template-movies.php
<?php /*Template Name: Template Movies*/ get_header(); $args =[ 'post_type' => 'movie', 'posts_per_page' => -1, ]; $movies = new WP_Query($args); ?> <main> <div class="movie_container" style="width: 80%; margin: 0 auto;"> <br> <div class="js-filter"> <?php $terms = get_terms(['taxonomy'=>'movie_type']); if($terms):?> <select id="cat" name="cat"> <option value="">Select Category</option> <?php foreach ($terms as $term): ?> <option value="<?php echo $term->slug; ?>"><?php echo $term->name; ?></option> <?php endforeach;?> </select> <?php endif; ?> <select name="popularity" id="popularity"> <option value="">Select Popularity</option> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> </select> </div> <?php if($movies->have_posts()): ?> <div class="js-movies row"> <?php while ($movies->have_posts()): $movies->the_post(); get_template_part('template-parts/loop','movie'); endwhile; ?> </div> <?php endif; ?> </div> </main> <?php get_footer(); ?>
Update following code in functions.php file
add_action( 'wp_enqueue_scripts', 'my_theme_enqueue_styles' ); function my_theme_enqueue_styles() { $parenthandle = 'parent-style'; // This is 'twentyfifteen-style' for the Twenty Fifteen theme. $theme = wp_get_theme(); wp_enqueue_style( $parenthandle, get_template_directory_uri() . '/style.css', array(), // if the parent theme code has a dependency, copy it to here $theme->parent()->get('Version') ); wp_enqueue_style( 'child-style', get_stylesheet_uri(), array( $parenthandle ), $theme->get('Version') // this only works if you have Version in the style header ); wp_enqueue_script('scripts-js',get_stylesheet_directory_uri().'/assets/js/scripts.js',['jquery'],'',true); wp_localize_script('scripts-js','variables',[ 'ajax_url' => admin_url('admin-ajax.php'), ]); } add_action('init','register_custom_post_types');
add wp_ajax in functions.php file
add_action('wp_ajax_filter_posts','filter_posts'); add_action('wp_ajax_nopriv_filter_posts','filter_posts'); function filter_posts(){ $args = [ 'post_type' => 'movie', 'posts_per_page' => -1, ]; $type = $_REQUEST['cat']; $rating = $_REQUEST['rating']; if(!empty($type)){ $args['tax_query'][] = [ 'taxonomy' => 'movie_type', 'field' => 'slug', 'terms' => $type, ]; } if(!empty($rating)){ $args['meta_query'][] = [ 'key' => 'rating', 'value' => $rating, 'compare' => '=', ]; } //echo "<pre>"; //print_r($args); //echo "</pre>"; $movies = new WP_Query($args); if($movies->have_posts()): while ($movies->have_posts()): $movies->the_post(); get_template_part('template-parts/loop','movie'); endwhile; wp_reset_postdata(); else: echo "Post Not Found"; endif; wp_die(); }
create javascript file for ajax call
twentytwenty-child/assets/js/scripts.js
jQuery(function ($) { $('.js-filter select').on('change',function () { var cat = $('#cat').val() rating = $('#popularity').val(); var data = { action: 'filter_posts', cat: cat, rating: rating, } $.ajax({ url: variables.ajax_url, type: 'POST', data: data, success: function (response) { //alert(response); $('.js-movies').html(response); } }) }); });
Leave a Reply