How to Get Featured Image URL in WordPress? If you’re making a WordPress theme, plugin, or something special, knowing how to show the featured image in WordPress can be really handy. You might want to show recent posts with a featured image or have it show up in your theme’s single post view. These functions will help you do that.
Keep in mind, if you want to use featured images in a theme, you’ll need to add the following code to your site’s functions.php file.
<?php //Enable theme support for featured images. add given code in functions.php add_theme_support('post-thumbnails');
In many themes, this feature is already turned on. But if you’re creating a theme from the beginning, you need to add support for post thumbnails. If you can see the option for a featured image in the WordPress editor, that means it’s already enabled.
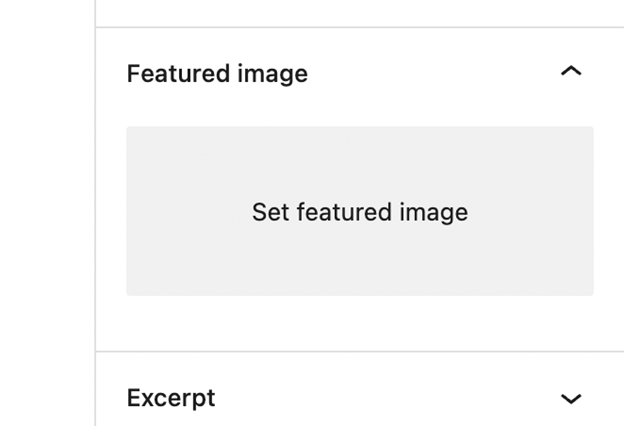
How to Display a Featured Image in PHP
To show a post’s featured image in an `<img>` tag, you can use the built-in WordPress function called `get_the_post_thumbnail()`. This is the simplest way to display a post’s featured image in a loop on WordPress.
<?php //Displays the featured image in a <img> tag (use this in a loop) echo get_the_post_thumbnail();
If you want to use a particular size for the featured image, you can also put in the name of the image size in the second part of the function.
<?php //Displays the featured image in a <img> tag resized to the 'large' thumbnail size (use this in a loop) echo get_the_post_thumbnail( get_the_ID(), 'large' );
Sometimes, you might want to use the web address (URL) of the featured image in your post. This is explained in the next part.
How to get WordPress Post Featured Image URL in PHP
If you have WordPress version 4.4 or newer (it came out in 2015), you can use a function called `get_the_post_thumbnail_url()` to get the web address (URL) of the featured image in your post. This is helpful if you want to use the image’s URL as a background image or for making something special in your theme that needs the image’s URL.
<?php //Display the featured post URL (you can replace 'medium' with a different image size) echo get_the_post_thumbnail_url( get_the_ID(), 'medium' );
You can also change the code above to work with different image sizes, like the ones that WordPress provides by default: thumbnail, medium, medium_large, large, and full.
How to get the Featured Image ID in PHP
With the `get_post_thumbnail_id()` function, you can find the unique ID of the featured image in a post. This is really useful when you want to use that ID for other things in WordPress.
<?php // Get the ID of the featured image echo get_the_post_thumbnail( get_the_ID(), 'large' );
I hope this information was useful for adding featured images on your WordPress site. If you have any questions, feel free to ask in the comments below!
Related Articles
Leave a Reply