In this tutorial, we’ll discuss image sizes in WordPress. But first, let’s clarify what image sizes are.
If you go to your WordPress “/wp-content/uploads” folder, you might see something like this:
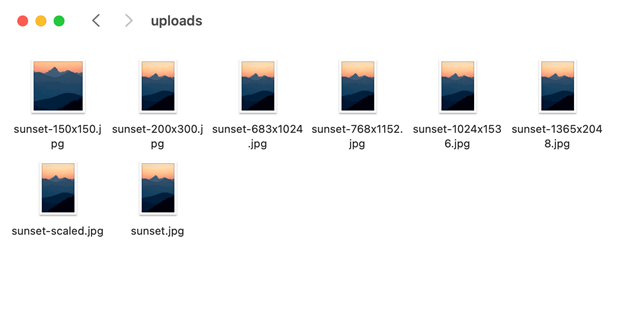
If you take a look, you’ll notice that when you upload an image once, WordPress creates multiple copies of it in different sizes. This happens even if you’re using the default WordPress theme without any additional plugins.
For people who aren’t familiar with this, it can be confusing to see so many duplicate images in the uploads directory.
Here’s the main idea: When you upload an original image, especially if it’s large and unoptimized (up to 10MB), it doesn’t make sense to use that same huge file everywhere on your website. You might need smaller versions for things like displaying recent posts with small 100×100 pixel thumbnails, and you also want to consider attributes like “srcset” and “sizes.” A practical example is the product list in WooCommerce’s admin area:
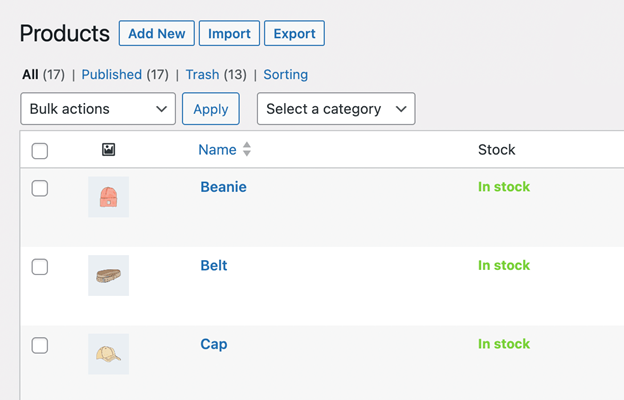
The images here are only about 50×50 pixels in size. Can you imagine what would happen to the time it takes for the webpage to load if we tried to use the original, larger images instead?
Of course, using image resizing software isn’t a good idea either because it can cause significant performance problems on your server.
That’s why WordPress makes various copies of images, and in this tutorial, we’ll learn how to handle and manage them effectively.
Let’s talk about the default image sizes in WordPress:
- Thumbnail: This is the size set in your WordPress settings under “Media.” It’s usually a small version of the image.
- Medium: Also set in your WordPress settings, it’s a medium-sized image.
- Medium Large: This size was introduced in WordPress 4.4 to support responsive images. It’s 768 pixels wide with a proportional height.
- Large: Another size set in your WordPress settings, and it’s larger than medium.
- 1536×1536 and 2048×2048: These sizes were added in WordPress 5.3. They are used to create better quality images for high-density screens.
- -scaled: This isn’t exactly a size but a version of an image that WordPress creates if the original image is very large (more than 2560 pixels in height or width). It gets the “-scaled” suffix.
- Full: This is the original, unaltered image.
You can control the sizes of thumbnail, medium, and large images in your WordPress settings under “Media.”
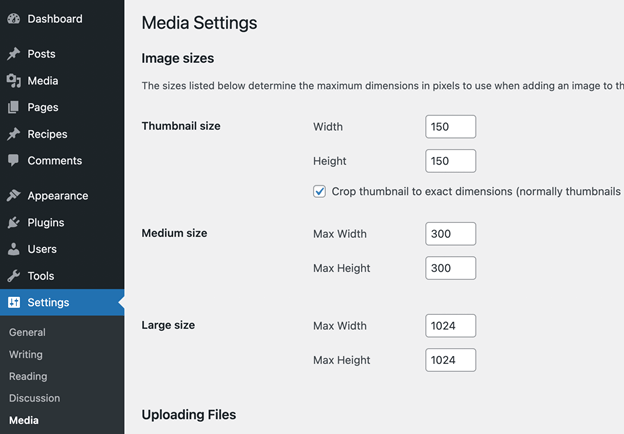
Registering Custom Image Sizes
1. add_image_size()
Creating a custom image size for your specific requirements is quite simple. You can do it by using the “add_image_size()” function in your functions.php file or any other suitable place. However, there are some details to consider, and we’ll talk about them shortly.
Here’s an example of how to register a custom image size:
add_action( 'after_setup_theme', function() { add_image_size( 'my-image-size-name', 200, 200, array( 'left', 'top' ) ); } );
Here are a couple of important things to remember:
1. It’s recommended to use the “add_image_size()” function inside the “after_setup_theme” hook, but it often works fine without it too.
2. The first three parameters of the function are pretty straightforward. The fourth argument is the most interesting one because it defines how images with different proportions should be cropped. In this example, I’m using a square size, so if the original image is wider than it is tall, the left part stays as is, but the right part gets cut off. For taller images, the bottom part is removed. However, you can set this argument to “false” if you don’t want cropping; instead, the images will only be resized. You can find more details about these function parameters in the official documentation.
3. Avoid creating too many custom image sizes because the more sizes you create, the more files will be stored in your uploads folder. This can slow down the process of uploading and managing images on your website.
2. Add your custom image size selection to Media Uploader and Block Editor
When you add images to your posts in WordPress, you can pick which version of the image size you want to use:
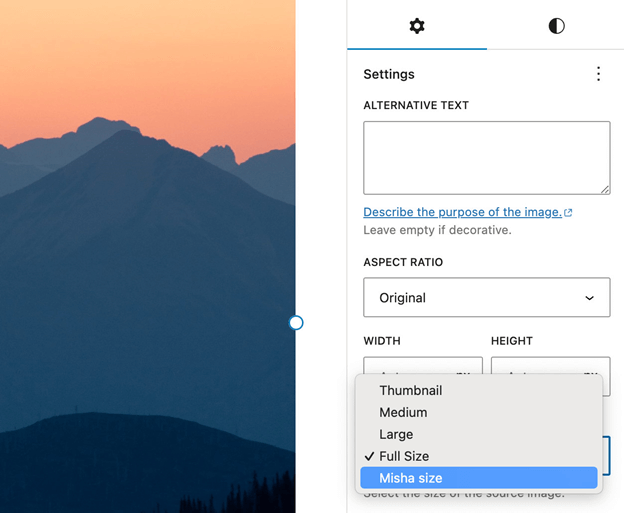
So, how can we make our custom image size show up there? Well, you can use the “image_size_names_choose” hook for that.
add_filter( 'image_size_names_choose', function( $sizes ) { // $sizes[ 'image size name' ] = 'Label (anything)'; $sizes[ 'my-image-size-name' ] = 'Diwakar size'; return $sizes; } );
3. Regenerate old media
The important thing to keep in mind is that when you create or remove image sizes, the new image files are generated only when you upload an image.
But do you have to re-upload images every time you make a change? Not at all! There are plenty of plugins designed for this purpose. For instance, I’ve used these ones:
1. AJAX Thumbnail Rebuild.
2. Force Regenerate Thumbnails.
However, in certain situations, you might want to regenerate thumbnails programmatically, and you can do that with the following function:
function dr_regenerate_sizes_for_image( $attachment_id ) { $path = get_attached_file( $attachment_id ); if( $path ) { wp_update_attachment_metadata( $attachment_id, wp_generate_attachment_metadata( $attachment_id, $path ) ); } }
Conditional image sizes registration – how to create certain image sizes for custom post types only?
Let’s imagine your website has 10 different types of content, and each of them uses 2-3 different image sizes on its pages. Now, when you upload a picture, WordPress creates 20-30 copies of that image to fit all these different sizes!
Here’s the tricky part: You can’t use the “add_image_size()” function to control this for custom post types. But there’s a clever piece of code that can help. This code tells WordPress when it should and shouldn’t create files for a specific image size.
You can use both the “intermediate_image_sizes” and “intermediate_image_sizes_advanced” hooks for this. Here’s a simple example: With this code, WordPress won’t create files for the “my-image-size-name” when you upload images for a custom post type page.
add_filter( 'intermediate_image_sizes_advanced', function( $sizes ){ $post_id = isset( $_REQUEST[ 'post_id' ] ) ? $_REQUEST[ 'post_id' ] : null; if( ! $post_id ) { return $sizes; } if( 'page' === get_post_type( $post_id ) ) { unset( $sizes[ 'my-image-size-name' ] ); } return $sizes; } );
Here’s a slightly more complex example, but it’s also correct:
add_filter( 'intermediate_image_sizes', function( $sizes ){ // $sizes - all the image sizes in an array // Array ( [0] => thumbnail [1] => medium [2] => large [3] => post-thumbnail ) $post_id = isset( $_REQUEST[ 'post_id' ] ) ? $_REQUEST[ 'post_id' ] : null; if( ! $post_id ) { return $sizes; } $post_type = get_post_type( $post_id ); foreach( $sizes as $key => $value ) { switch( $post_type ) { case 'regionfeatured' : { unset( $sizes[ $key ] ); break; } case 'region' : { if( ! in_array( $value, array( 'regionfeatured', 'misha335' ) ) ) { unset( $sizes[ $key ] ); } breal; } default : { // turn off everything except thumbnail if( 'thumbnail' !== $value ){ unset( $sizes[$key] ); } break; } } } return $sizes; }
Removing Image Sizes
Of course, in some cases, you don’t need so many image files. For example, this website doesn’t use any!
So, how can you get rid of them?
First, you can set all the image sizes to 0 in the settings, like this:
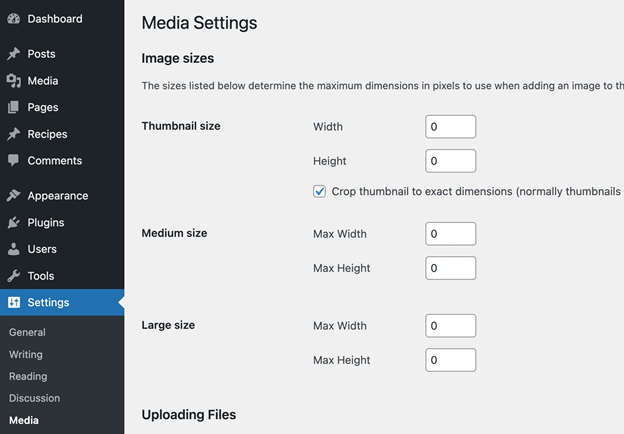
Alternatively, you can use the “intermediate_image_sizes” filter hook. You can also use the “remove_image_size()” function inside the “after_setup_theme” hook, similar to how you registered them in the first place.
add_filter( 'intermediate_image_sizes', 'dr_turn_off_default_sizes' ); function dr_turn_off_default_sizes( $sizes ) { unset( $sizes[ 'thumbnail' ] ); unset( $sizes[ 'medium' ] ); unset( $sizes[ 'large' ] ); unset( $sizes[ 'medium_large' ] ); // remove medium_large image size unset( $sizes[ '1536x1536' ] ); // remove 1536x1536 image size unset( $sizes[ '2048x2048' ] ); // remove 2048x2048 image size return $sizes; }
When dealing with images that have the “-scaled” suffix, the approach is a bit different.
// remove -scaled image sizes add_filter( 'big_image_size_threshold', '__return_false' );
Related Articles
Leave a Reply