You might want to find a user’s ID in WordPress for various purposes. Whether it’s for custom code or troubleshooting, this guide will explain how to locate user IDs in WordPress.
We’ll start by exploring methods to find user IDs within the WordPress dashboard. Later in the article, we’ll also delve into using PHP code snippets to retrieve user IDs.
Here are five methods you can use to find a user’s ID in WordPress:
Find User ID in WordPress Dashboard
This is the easiest way to get a user’s ID in WordPress. To start, log in to your WordPress admin dashboard. After that, navigate to the “Users” section and click on “All Users.”
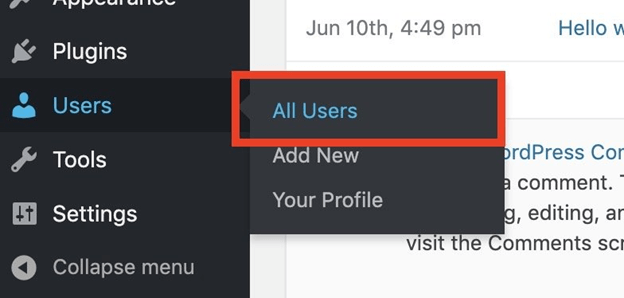
From this point, you can choose the user for whom you want to find the ID. Just click on the “Edit” option next to their name.
This will take you to the page where you can edit the user’s information. Here, you can find the user’s ID in the web address at the top of your browser.
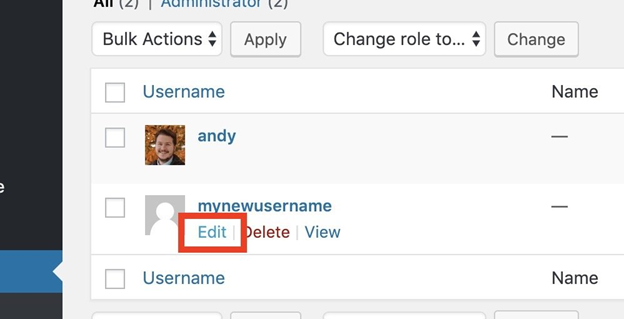
For instance, in the example below, the user’s ID is “3”.
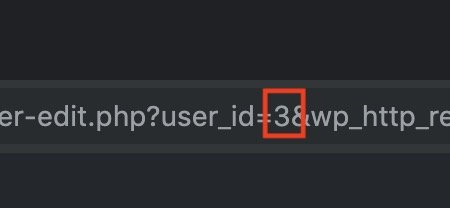
See All WordPress User IDs (Using Reveal IDs Plugin)
If you want to view the IDs of all users in your WordPress dashboard, you can use a plugin called “Reveal IDs.” After you activate this plugin, simply go to “Users” and then “All Users” in your dashboard. You’ll notice a new column displaying the user IDs.
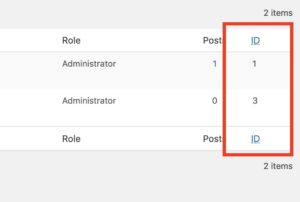
This plugin is a useful tool for showing user IDs right in the admin area of WordPress, making it easy to reference them quickly.
Get Current User ID (using PHP)
The simplest method to retrieve the ID of the currently logged-in user using PHP is by using the function called get_current_user_id(). This is especially useful if you’re creating custom code and require the ID of the user who is currently logged in.
<?php echo 'The current logged in user ID is: '.get_current_user_id();
If you need more than just the user ID, you can use the function wp_get_current_user(). This function provides you with an object containing various details about the currently logged-in user. For instance, you can use it to retrieve the user’s ID, username, full name, display name, and email address.
<?php $current_user = wp_get_current_user(); echo 'Username: '.$current_user->user_login; echo 'User ID: '.$current_user->ID; echo 'User Email: '.$current_user->user_email; echo 'User First Name: '.$current_user->user_firstname; echo 'User Last Name: '.$current_user->user_lastname; echo 'User Display Name: '.$current_user->display_name;
Get User ID by Email (using PHP)
If you want to find a WordPress user’s ID using their email address, you can achieve this using the get_user_by() function. This function allows you to search for a user based on their email address and retrieve their corresponding user ID.
<?php //Get user ID by email $user_data = get_user_by('email', '[email protected]'); $user_id = $user_data->ID; $user_email = $user_data->user_email; if(!empty($user_id)){ echo 'The user ID for '.$user_email.' is '.$user_id; }
Get User ID by Username (using PHP)
If you’re aiming to obtain a WordPress user’s ID using their username, the most effective approach is to utilize the get_user_by() function. This function enables you to locate a user based on their username and retrieve their associated user ID.
<?php $user_data = get_user_by('login', 'someguy'); echo 'The user ID is '.$user_data->ID;
Best wishes as you embark on your WordPress development journey! I hope these simple tips for finding a user’s ID in WordPress help you resolve any concerns you might have. If you have any inquiries, feel free to share them in the comments section below!
Related Articles
Leave a Reply