Today, some people might think that WordPress Meta Boxes are no longer important because of Gutenberg and Full Site Editing. But I want to tell you that’s not entirely true.
Even though Gutenberg is getting better and more plugins are available for custom fields, it’s still valuable to know how to create a metabox from the beginning. For example, if you take a look at WooCommerce, their Products section doesn’t use Gutenberg yet. In some cases, you might need a post type that doesn’t require visual editing, and that’s when meta boxes become useful.
What are Meta Boxes Exactly?
Meta boxes are like special boxes in the WordPress admin area that help you control extra information about your posts.
Now, what’s this “extra information” called metadata?
In WordPress, all different types of content, like posts, categories, users, and comments, have their own place in the WordPress database. It’s like a giant storage space with separate areas for each type of content. These storage areas are called wp_posts, wp_terms, wp_users, and wp_comments. They are designed to keep basic information like post titles and publish dates.
But what if you want to save something really specific? Let’s say you want to save an SEO title for your posts, or an age for users, or even the price of a product. That’s when metadata comes into play. WordPress has special places for this kind of information in the database, too. They’re called wp_postmeta, wp_termmeta, wp_usermeta, and wp_commentmeta. Here’s what it looks like when you check it out in phpMyAdmin:
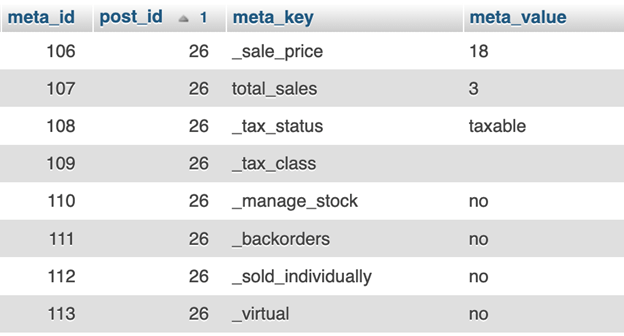
Table Name: wp_postmeta
Meta boxes help you control this data, like in WooCommerce. You can notice that the value you see as “_sale_price” in the table above is the same as what’s in the “Sale price” field below.
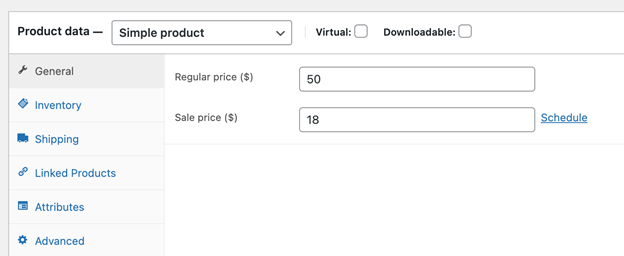
Product data metabox in WooCommerce
How to Remove Default Meta Boxes
Every time you’re editing a post in WordPress, you’ll find some boxes already there with important info. If you’re using the “Classic editor” plugin, these boxes are visible.
Now, if you want to get rid of one of these boxes, there are two simple ways to do it. You can either use the “remove_meta_box()” function or use “remove_post_type_support().
remove_meta_box()
Let’s jump right into an example. Imagine we want to get rid of two boxes: “Page Attributes” for pages and “Custom Fields” for posts. Here’s the code to do that:
add_action( 'admin_menu', 'da_remove_meta_box' ); function da_remove_meta_box(){ // Custom Fields remove_meta_box( 'postcustom', 'post', 'normal' ); // Page Attributes remove_meta_box( 'pageparentdiv', 'page', 'normal' ); }
In WordPress, there are different boxes on the editing pages for posts and pages. These boxes help you do specific things. Here are some examples:
– “Discussion” helps with comments.
– “Slug” is for the web address.
– “Comments” manages comments.
– “Excerpt” is a short summary.
– “Author” is about the post’s author.
– “Revisions” tracks changes.
– “Custom Fields” is for special info.
– “Send Trackbacks” deals with links.
– “Categories” organizes posts.
– “Tags” adds keywords.
– “Any Taxonomy” is for custom categories.
– “Featured Image” can’t be removed with “remove_meta_box()” function.
– “Page Attributes” is for page settings.
– “Publish” is for posting.
Now, if you want to get rid of one of these boxes for a specific type of content (like posts or pages), you can use a code function called “remove_meta_box().” You tell it which box you want to remove and for which type of content.
But there’s another way. Instead of removing the whole box, you can disable just one part of it using “remove_post_type_support().” The cool thing is, this method works for both the new Gutenberg editor and the older Classic Editor.
add_action( 'init', 'diwakar_remove_meta_box_2' ); function diwakar_remove_meta_box_2() { // Featured image remove_post_type_support( 'page', 'thumbnail' ); // Page attributes remove_post_type_support( 'page', 'page-attributes' ); }
I believe the best way to get rid of default WordPress boxes is by using the “remove_post_type_support()” function. It’s better because it actually turns off a specific feature for a certain type of content. On the other hand, “remove_meta_box()” just gets rid of a box but doesn’t disable the feature.
These are the features (or meta boxes) you can control:
– “thumbnail” — The Featured Image box.
– “author” — The Author box.
– “excerpt” — The Excerpt box.
– “trackbacks” — The Send Trackbacks box.
– “custom-fields” — The Custom Fields box.
– “comments” — The Comments box.
– “revisions” — The Revisions box.
– “page-attributes” — The Page Attributes box (this one is for Gutenberg only).
You can also remove parts like the title or the content editor:
– “title”
– “editor”
Now, in the previous section, we didn’t talk about removing boxes for taxonomies (like categories or tags). That’s because you can’t use “remove_post_type_support()” for taxonomies, whether they’re custom or not.
But don’t worry, there’s another way to handle that:
add_action( 'init', 'diwakar_remove_taxonomy_meta_boxes' ); function diwakar_remove_taxonomy_meta_boxes() { // for Post tags unregister_taxonomy_for_object_type( 'post_tag', 'post' ); // for Categories unregister_taxonomy_for_object_type( 'category', 'post' ); }
Just remember, this method completely separates a particular category from a type of content. If you only want to get rid of the category box but keep them connected, it’s better to use a different method.
Add Custom Meta Box
We’ve reached the part of the tutorial where I’ll teach you how to make a special box in WordPress. Check out this picture to see what we’re going to create:
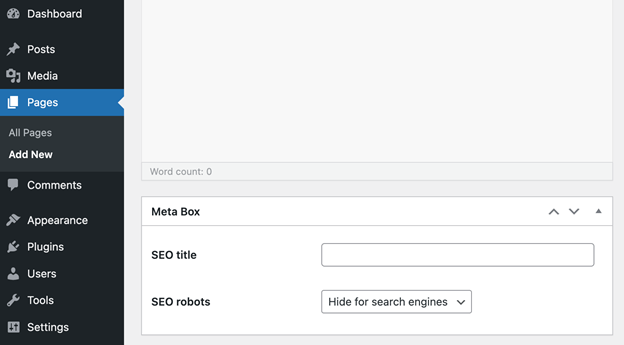
WordPress custom meta box with multiple fields for “Pages” post type.
Step 1. add_meta_box()
To use the “add_meta_box()” function, you should put it inside something called an action hook. There are three choices:
1. “admin_menu”
2. “add_meta_boxes”
3. “add_meta_boxes_{post_type}”
The third one, “add_meta_boxes_{post_type}”, lets you pick a certain type of content, like a page. So, the code you write will only work when you’re editing that specific type of content.
add_action( 'admin_menu', 'diwakar_add_metabox' ); // or add_action( 'add_meta_boxes', 'diwakar_add_metabox' ); // or add_action( 'add_meta_boxes_{post_type}', 'diwakar_add_metabox' ); function diwakar_add_metabox() { add_meta_box( 'diwakar_metabox', // metabox ID 'Meta Box', // title 'diwakar_metabox_callback', // callback function 'page', // add meta box to custom post type (or post types in array) 'normal', // position (normal, side, advanced) 'default' // priority (default, low, high, core) ); }
// it is a callback function which actually displays the content of the meta box
function diwakar_metabox_callback( $post ) { echo 'hey'; }
After you put this code into the functions.php file of your child theme or a custom plugin, you’ll see this new box show up:
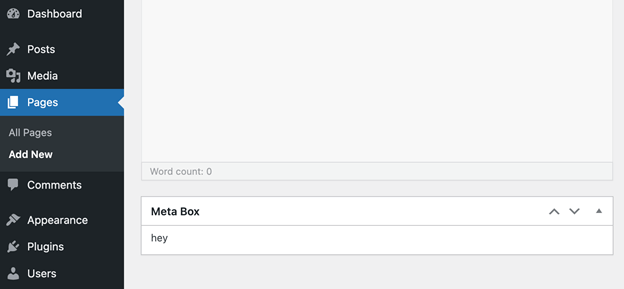
Here’s a simple example of how to use the “add_meta_box()” function.
When you look at the box, you’ll notice that everything we put in the callback function now shows up inside the box.
Now, let’s go deeper and add some fields to our box.
In the code below, I added several fields to our special box.
In the code above:
1. I used the “get_post_meta()” function to grab the values of existing meta fields and show them.
2. We have “wp_nonce_field()” for added security. When we save our meta box data, we can double-check if the request is from the right source by checking this nonce value. 3. There’s a handy built-in WordPress function called “selected()” that makes it easier to decide which option should be marked as “selected” in a dropdown list (<select> field).
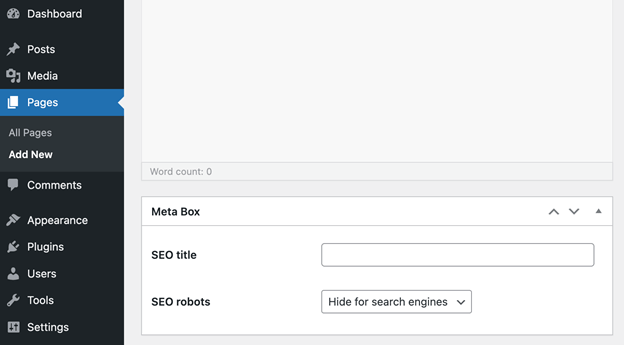
Step 3. Save meta box data
If you’re using this special box on the page editing screen or for a custom content type, make sure you specify which content types it should work with on line 23. If you skip this step, the information in the box won’t be saved properly.
The functions “update_post_meta()” and “delete_post_meta()” (mentioned in lines 27-36) should be used for each input field you have in your special box. They help save and delete the information you put in those fields.
add_action( 'save_post', 'diwakar_save_meta', 10, 2 ); // or add_action( 'save_post_{post_type}', 'diwakar_save_meta', 10, 2 ); function diwakar_save_meta( $post_id, $post ) { // nonce check if ( ! isset( $_POST[ '_diwakarnonce' ] ) || ! wp_verify_nonce( $_POST[ '_diwakarnonce' ], 'somerandomstr' ) ) { return $post_id; } // check current user permissions $post_type = get_post_type_object( $post->post_type ); if ( ! current_user_can( $post_type->cap->edit_post, $post_id ) ) { return $post_id; } // Do not save the data if autosave if ( defined('DOING_AUTOSAVE') && DOING_AUTOSAVE ) { return $post_id; } // define your own post type here if( 'page' !== $post->post_type ) { return $post_id; } if( isset( $_POST[ 'seo_title' ] ) ) { update_post_meta( $post_id, 'seo_title', sanitize_text_field( $_POST[ 'seo_title' ] ) ); } else { delete_post_meta( $post_id, 'seo_title' ); } if( isset( $_POST[ 'seo_robots' ] ) ) { update_post_meta( $post_id, 'seo_robots', sanitize_text_field( $_POST[ 'seo_robots' ] ) ); } else { delete_post_meta( $post_id, 'seo_robots' ); } return $post_id; }
Remember, depending on the type of information you’re dealing with in your fields, you need to make sure it’s clean and safe to use. In the example above, I’m using a function called “sanitize_text_field()” to do that. But if you have different types of fields, like a bigger text area, there are other functions like “sanitize_textarea()” or “sanitize_email()” to use.
Also, you don’t always have to use “delete_post_meta()” for every field. For regular text fields, it’s not necessary. However, it’s a good idea to check if a field has a value using “isset()” or “empty()” to avoid getting PHP notices if the field is empty. This helps keep things smooth and error-free.
$seo_title = isset( $_POST[ 'seo_title' ] ) ? sanitize_text_field( $_POST[ 'seo_title' ] ) : ''; update_post_meta( $post_id, 'seo_title', $seo_title );
Gutenberg Compatibility Flags
Normally, when you create a special box, it shows up in both the classic editor and the new Gutenberg editor. That’s okay sometimes, but you should know that this happens to make sure old stuff still works with the new editor. Ideally, you wouldn’t want these boxes to appear in Gutenberg.
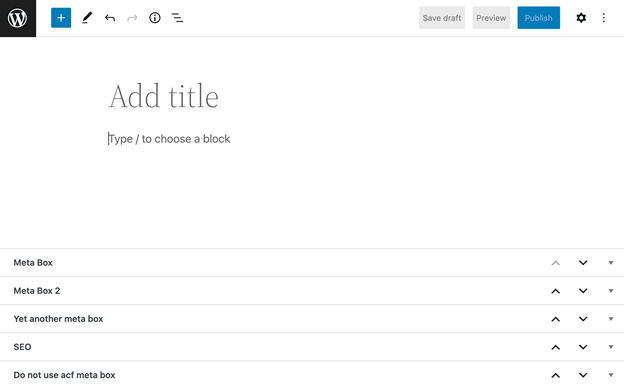
But what if you’re not sure if your users will use Gutenberg or the Classic Editor? You can use two flags (like settings) when you create your special box to help with that.
– “__back_compat_meta_box”: If you set this to “true,” your box will only show up in the Classic Editor, not Gutenberg.
– “__block_editor_compatible_meta_box”: If you set this to “false,” in Gutenberg, it will tell users that your box doesn’t work with the block editor and suggests using the Classic Editor instead.
Here’s an example using “__back_compat_meta_box:”
add_meta_box( 'diwakar_metabox', 'Meta Box', 'diwakar_metabox_callback', 'page', 'normal', 'default', array( '__back_compat_meta_box' => true, ) );
Example of using __block_editor_compatible_meta_box:
add_meta_box( 'diwakar_metabox', 'Meta Box', 'diwakar_metabox_callback', 'page', 'normal', 'default', array( '__block_editor_compatible_meta_box' => false, ) );
That’s the message in Gutenberg:
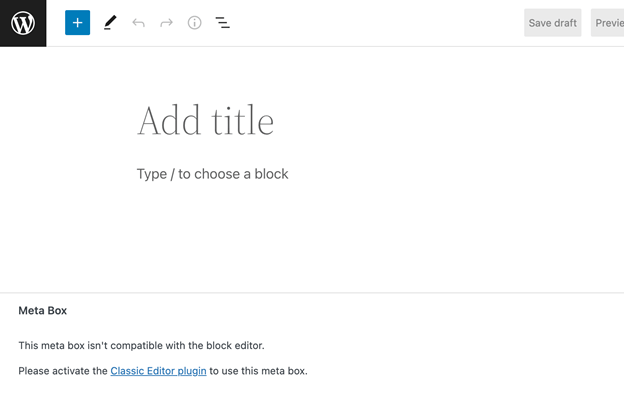
Flag __block_editor_compatible_meta_box
is set to false
.
Related Articles
Leave a Reply